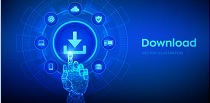
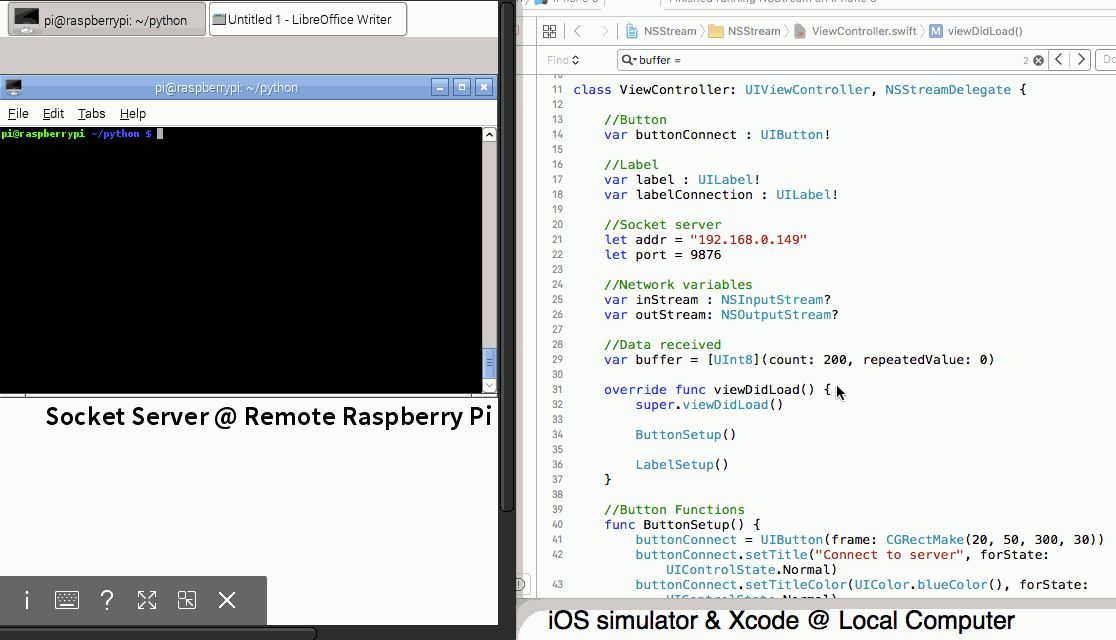

mkd(_directory_) will create a new directory.For instance, rename ('spam.txt', 'eggs.txt') will rename the file spam.txt, but rename('spam.txt', 'new_directory/spam.txt') will move spam.txt into the new_directory within the present directory. rename(_old filename_, _new filename_) will rename a file in the same directory, or move a file to a new directory, if you specify a new path in the second argument.delete(_filename_) will delete a file from the server.Now you should be logged in, and you can experiment with some of the following library calls: > ftp.login('_your_username_', '_your_password_') To get a demonstration, connect to your FTP server: > from ftplib import FTP Like most library calls, you can experiment with these using an interactive Python prompt, rather than a complete, standalone script. These can be somewhat obscure calls since they’re not used very often, but they are very straightforward. Like any good FTP client, the ftplib library supports file deletion, renaming, moving, and even directory creation and deletion. Should you want to copy a text file instead, the script should look something like this: from ftplib import FTPįtp.storlines('STOR %s' % 'remotefile.txt', f) This should have the effect of copying myfile.py from your local directory to the remote directory on your FTP server. Once you have access to an FTP server, open a text editor and enter the following Python script: from ftplib import FTPįtp.storbinary('STOR %s' % 'remotefile.py', f) A nice feature of these functions is that neither one requires you to write a separate function to handle reading the source file: storlines() calls the readline() method on each line in the file until it reads the last line, while storbinary() uses the read() method until there is no more data to read and upload. You can upload text files using the storlines() method and binary files with the storbinary() method.
#Python connect to ftp server install
Assuming you do not have access to a web-based FTP server, your best bet is to install a server on your local machine (see “ Installing an FTP server“) and test your code using localhost as the target server.Īs with downloads, you’ll need to specify whether a file you wish to upload to a server is a text file or a binary file since each uses a different method. It is difficult to experiment with many of these calls with a server that you don’t own–most FTP servers will not allow anonymous logins. In this segment, I’ll introduce several new concepts, including uploading text and binary files, error handling, and common directory commands using the same imported library.
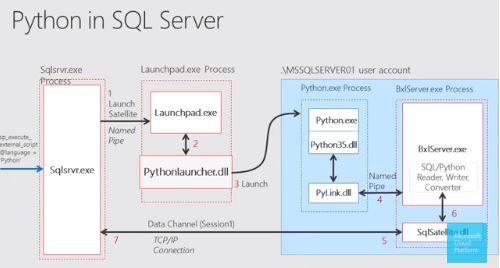
#Python connect to ftp server download
In another article on using ftplib in Python, we talked about using Python’s ftplib library to connect to an FTP server and download both binary and text files to our local machine.
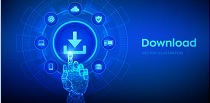